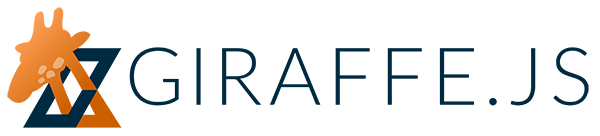
View UI
This example demonstrates the Giraffe.View ui
feature that maintains
cached jQuery objects for a view and allows you to use the names of these
cached objects in your events
hash. The ui
hash is a simple map of names to
selectors. For example, {$button: 'button'}
makes this.$button
available
once the view has rendered at least once.
var View = Giraffe.View.extend({
ui: {
'$someButton': 'button'
},
events: {
'click $someButton': function() {
alert('clicked `this.$someButton` which has length ' + this.$someButton.length);
}
},
template: '#view-template'
});
<script id="view-template" type="text/template">
<button>click me</button>
</script>
Let's create and attach the view to see it in action.
var view = new View();
view.attachTo('body');
Try It
var View = Giraffe.View.extend({
ui: {
'$someButton': 'button'
},
events: {
'click $someButton': function() {
alert('clicked `this.$someButton` which has length ' + this.$someButton.length);
}
},
template: '#view-template'
});
var view = new View();
view.attachTo('body');
<!DOCTYPE html>
<html>
<head>
<link rel='stylesheet' type='text/css' href='../css/reset.css' />
</head>
<body>
<script id="view-template" type="text/template">
<button>click me</button>
</script>
<script src="http://code.jquery.com/jquery-1.9.1.min.js"></script>
<script src="http://cdnjs.cloudflare.com/ajax/libs/underscore.js/1.4.4/underscore-min.js"></script>
<script src="http://cdnjs.cloudflare.com/ajax/libs/backbone.js/1.0.0/backbone-min.js"></script>
<script src="../backbone.giraffe.js" type="text/javascript"></script>
<script type='text/javascript' src='viewui0-script.js'></script>
</body>
</html>