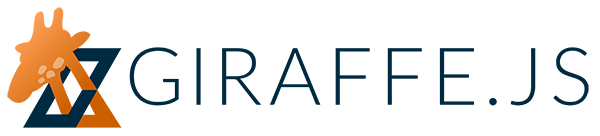
Lifecycle Management
This example demonstrates the lifecycle management features in Giraffe.
All Giraffe objects implement a dispose
method. When a Giraffe.View is
disposed, it calls dispose
on all of its children
that have the method. In
this example we'll create a Giraffe.App, give it some children, dispose
of
it, and see the results.
var app = new Giraffe.App();
app.attachTo('body');
Giraffe.App, which is a special Giraffe.View, is designed to encapsulate
an entire application, but for the purposes of this example we're using no
features specific to it - a Giraffe.View would have worked too.
Let's listen to the built-in disposal events and write out what's happening.
app.once('disposing', function() {
$('body').append('<p>app is disposing</p>');
});
app.once('disposed', function() {
$('body').append('<p>app is disposed</p>');
});
The
Giraffe.View#dispose
method overrides the behavior of
Backbone.View#remove
function. We didn't want to rename it, but because we
want Giraffe to manage the lifecycle of any object, and remove
means
something different for collections, we opted to use the method name dispose
.
Now that the app is ready, let's give it some children.
var childModel = new Giraffe.Model();
app.addChild(childModel);
var childCollection = new Giraffe.Collection([{'name': 'a model!'}]);
app.addChild(childCollection);
var childView = new Giraffe.View();
app.attach(childView);
// `app.addChild(childView)` also works, but doesn't put childView.$el in app.$el
To help us follow the action of dispose
, we'll listen for the events signaling
when these objects are disposed and write out what's happening.
childModel.once('disposed', function() {
$('body').append('<p>model is disposed</p>');
});
childCollection.once('disposed', function() {
$('body').append('<p>collection has ' + this.length + ' models</p>');
$('body').append('<p>collection is disposed</p>');
});
childCollection.models[0].once('disposed', function() {
$('body').append('<p>collection\'s model is disposed</p>');
});
childView.once('disposed', function() {
$('body').append('<p>view is disposed</p>');
});
Giraffe.Collection and Giraffe.Model are very thin wrappers over their
Backbone counterparts, adding only
dispose
and appEvents
support. They
are by no means required, and you can have Giraffe manage the lifecycles of
any objects with a dispose
method. If you want to reuse the same dispose
method Giraffe's classes use, it's available at Giraffe.dispose
. It calls
stopListening
, triggers the 'disposing'
and 'disposed'
events, and sets
this.app
to null
. It also accepts a function argument to do additional work.
Any object with a dispose
method can be added to a view's children
to be
cleaned up.
var someObject = {
dispose: function() {
Giraffe.dispose.call(this);
$('body').append('<p>someObject is disposed</p>');
}
};
app.addChild(someObject);
Let's call dispose
on the app and see what happens!
app.dispose();
Here's what happened:
var app = new Giraffe.App();
app.attachTo('body');
app.once('disposing', function() {
$('body').append('<p>app is disposing</p>');
});
app.once('disposed', function() {
$('body').append('<p>app is disposed</p>');
});
var childModel = new Giraffe.Model();
app.addChild(childModel);
var childCollection = new Giraffe.Collection([{
'name': 'a model!'
}]);
app.addChild(childCollection);
var childView = new Giraffe.View();
app.attach(childView);
// `app.addChild(childView)` also works, but doesn't put childView.$el in app.$el
childModel.once('disposed', function() {
$('body').append('<p>model is disposed</p>');
});
childCollection.once('disposed', function() {
$('body').append('<p>collection has ' + this.length + ' models</p>');
$('body').append('<p>collection is disposed</p>');
});
childCollection.models[0].once('disposed', function() {
$('body').append('<p>collection\'s model is disposed</p>');
});
childView.once('disposed', function() {
$('body').append('<p>view is disposed</p>');
});
var someObject = {
dispose: function() {
Giraffe.dispose.call(this);
$('body').append('<p>someObject is disposed</p>');
}
};
app.addChild(someObject);
app.dispose();
<!DOCTYPE html>
<html>
<head>
<link rel='stylesheet' type='text/css' href='../css/reset.css' />
</head>
<body>
<script src="http://code.jquery.com/jquery-1.9.1.min.js"></script>
<script src="http://cdnjs.cloudflare.com/ajax/libs/underscore.js/1.4.4/underscore-min.js"></script>
<script src="http://cdnjs.cloudflare.com/ajax/libs/backbone.js/1.0.0/backbone-min.js"></script>
<script src="../backbone.giraffe.js" type="text/javascript"></script>
<script type='text/javascript' src='lifecyclemanagement0-script.js'></script>
</body>
</html>