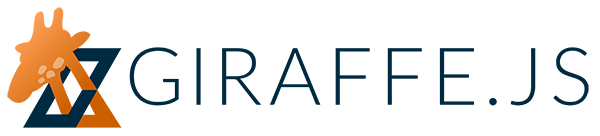
View Data Events
This example demonstrates how to use the dataEvents
map of Giraffe.View.
Similar to how the Backbone.View events
map binds DOM events to view
methods, Giraffe.View provides the dataEvents
hash that maps object events
to view methods. Like the events
map, the dataEvents
bindings are also
automatically cleaned up when a view's dispose
method is called.
var View = Giraffe.View.extend({
To demonstrate dataEvents
, we'll first need some data. In this example we'll
use a regular Giraffe.Collection, but dataEvents
works with any object
that implements Backbone.Events.
initialize: function() {
this.collection = new Giraffe.Collection();
},
dataEvents
maps events on an object to a view method via Backbone.Events#listenTo
.
Its key is a space-separated series of events ending with the target object.
This structure mirrors the events
map of Backbone.View,
{'domEventName selector': 'viewMethod'}
, but replaces the selector with the
name of any Backbone.Events
object on this view instance.
As a result of using listenTo
, dataEvents
accepts multiple events per
definition and the handlers are called in the context of the view.
dataEvents: {
'add remove collection': 'render'
// 'someEvent anotherEvent someBackboneEventsObject': function() { ... }
// 'anEventTriggeredOnThisView this': 'someMethodName' // listen to self
// 'sameEventAsAbove @': 'sameMethodAsAbove'
},
this.listenTo(this.collection, 'add remove', this.render);
in initialize
.
This example has a button to add a new model and a button for each model that removes it. The Document Events feature is used to bind click events to view methods.
template: '#view-template',
<script id="view-template" type="text/template">
<button data-gf-click="onAddModel">add model</button>
<% collection.each(function(model, index) { %>
<button data-gf-click="onRemoveModel" data-cid="<%= model.cid %>">
remove model <%= model.cid %>
</button>
<% }); %>
</script>
Here are the functions that add and remove models. The ui updates automatically
on the 'add'
and 'remove'
events bound in dataEvents
.
onAddModel: function(e) {
this.collection.add({});
},
onRemoveModel: function(e) {
var cid = $(e.target).data('cid');
this.collection.remove(cid);
}
});
That's it! Let's create and attach the view.
var view = new View();
view.attachTo('body');
dataEvents
fails for some use cases. Its events are bound
directly after Giraffe.View#initialize
, so if your view needs to respond
to events in the constructor
or initialize
, they won't yet be listened
for, and if your view creates data objects after initialize
, they won't
be bound to. We advocate using Backbone.Events#listenTo
directly in these
circumstances.
Try It
var View = Giraffe.View.extend({
initialize: function() {
this.collection = new Giraffe.Collection();
},
dataEvents: {
'add remove collection': 'render'
// 'someEvent anotherEvent someBackboneEventsObject': function() { ... }
// 'anEventTriggeredOnThisView this': 'someMethodName' // listen to self
// 'sameEventAsAbove @': 'sameMethodAsAbove'
},
template: '#view-template',
onAddModel: function(e) {
this.collection.add({});
},
onRemoveModel: function(e) {
var cid = $(e.target).data('cid');
this.collection.remove(cid);
}
});
var view = new View();
view.attachTo('body');
<!DOCTYPE html>
<html>
<head>
<link rel='stylesheet' type='text/css' href='../css/reset.css' />
</head>
<body>
<script id="view-template" type="text/template">
<button data-gf-click="onAddModel">add model</button>
<% collection.each(function(model, index) { %>
<button data-gf-click="onRemoveModel" data-cid="<%= model.cid %>">
remove model <%= model.cid %>
</button>
<% }); %>
</script>
<script src="http://code.jquery.com/jquery-1.9.1.min.js"></script>
<script src="http://cdnjs.cloudflare.com/ajax/libs/underscore.js/1.4.4/underscore-min.js"></script>
<script src="http://cdnjs.cloudflare.com/ajax/libs/backbone.js/1.0.0/backbone-min.js"></script>
<script src="../backbone.giraffe.js" type="text/javascript"></script>
<script type='text/javascript' src='dataevents0-script.js'></script>
</body>
</html>